fastmybatis 2.8.1 发布,支持JPA Query Method查询
fastmybatis 2.8.1 发布,本次更新内如下:
- 新增JPA Query Method查询
原理:根据方法名称中的关键字自动推导出SQL语
目前实现了大部分功能,参考: JPA Query Method 除了Distinct不支持,其它已全部支持
在Mapper中定义一个方法,以 findBy 开头
/** * … where x.lastname = ?1 and x.firstname = ?2 * @param lastname * @param firstname * @return */ List<Student> findByLastnameAndFirstname(String lastname, String firstname);
使用:
@Test public void findByLastnameAndFirstname() { List<Student> users = mapper.findByLastnameAndFirstname("张", "三"); Assert.assertEquals(1, users.size()); users.forEach(System.out::println); }
And | findByLastnameAndFirstname | … where x.lastname = ?1 and x.firstname = ?2 |
Or | findByLastnameOrFirstname | … where x.lastname = ?1 or x.firstname = ?2 |
Is , Equals | findByFirstname ,findByFirstnameIs ,findByFirstnameEquals | … where x.firstname = ?1 |
Between | findByStartDateBetween | … where x.startDate between ?1 and ?2 |
LessThan | findByAgeLessThan | … where x.age < ?1 |
LessThanEqual | findByAgeLessThanEqual | … where x.age <= ?1 |
GreaterThan | findByAgeGreaterThan | … where x.age > ?1 |
GreaterThanEqual | findByAgeGreaterThanEqual | … where x.age >= ?1 |
After | findByStartDateAfter | … where x.startDate > ?1 |
Before | findByStartDateBefore | … where x.startDate < ?1 |
IsNull , Null | findByAge(Is)Null | … where x.age is null |
IsNotNull , NotNull | findByAge(Is)NotNull | … where x.age not null |
Like | findByFirstnameLike | … where x.firstname like '%?1%' |
NotLike | findByFirstnameNotLike | … where x.firstname not like '%?1%' |
StartingWith | findByFirstnameStartingWith | … where x.firstname like '?1%' |
EndingWith | findByFirstnameEndingWith | … where x.firstname like '%?1' |
Containing | findByFirstnameContaining | … where x.firstname like '%?1%' |
OrderBy | findByAgeOrderByLastnameDesc | … where x.age = ?1 order by x.lastname desc |
Not | findByLastnameNot | … where x.lastname <> ?1 |
In | findByAgeIn(Collection<Age> ages) | … where x.age in ?1 |
NotIn | findByAgeNotIn(Collection<Age> ages) | … where x.age not in ?1 |
True | findByActiveTrue() | … where x.active = true |
False | findByActiveFalse() | … where x.active = false |
IgnoreCase | findByFirstnameIgnoreCase | … where UPPER(x.firstname) = UPPER(?1) |
完整测试用例:
/** * 演示JPA findBy查询,根据方法名称自动生成查询语句,无需编写SQL * @author tanghc */ public interface StudentMapper extends CrudMapper<Student, Integer> { /** * … where x.lastname = ?1 and x.firstname = ?2 * @param lastname * @param firstname * @return */ List<Student> findByLastnameAndFirstname(String lastname, String firstname); /** * * … where x.lastname = ?1 or x.firstname = ?2 * @param lastname * @param firstname * @return */ List<Student> findByLastnameOrFirstname(String lastname, String firstname); /** * where x.firstname = ? * @param firstname * @return */ List<Student> findByFirstname(String firstname); List<Student> findByFirstnameIs(String firstname); List<Student> findByFirstnameEquals(String firstname); /** * … where x.startDate between ?1 and ?2 * @param begin * @param end * @return */ List<Student> findByStartDateBetween(Date begin, Date end); /** * … where x.age < ?1 * @param age * @return */ List<Student> findByAgeLessThan(int age); /** * … where x.age <= ?1 * @param age * @return */ List<Student> findByAgeLessThanEqual(int age); /** * … where x.age > ?1 * @param age * @return */ List<Student> findByAgeGreaterThan(int age); /** * … where x.age >= ?1 * @param age * @return */ List<Student> findByAgeGreaterThanEqual(int age); /** * … where x.startDate > ?1 * @param date * @return */ List<Student> findByStartDateAfter(Date date); /** * … where x.startDate < ?1 * @param date * @return */ List<Student> findByStartDateBefore(Date date); /** * … where x.age is null * @return */ List<Student> findByAgeNull(); List<Student> findByAgeIsNull(); /** * … where x.firstname like ?1 * @param firstname * @return * @see #findByFirstnameContaining(String) */ List<Student> findByFirstnameLike(String firstname); /** * … where x.firstname not like ?1 * @param firstname * @return */ List<Student> findByFirstnameNotLike(String firstname); /** * … where x.firstname like 'xx%' * @param firstname * @return */ List<Student> findByFirstnameStartingWith(String firstname); /** * … where x.firstname like '%xx' * @param firstname * @return */ List<Student> findByFirstnameEndingWith(String firstname); /** * 等同于like * … where x.firstname like '%xx%' * @param firstname * @return */ List<Student> findByFirstnameContaining(String firstname); /** * … where x.age = ?1 order by x.lastname desc * @param age * @return */ List<Student> findByAgeOrderByLastnameDesc(int age); /** * … where x.lastname <> ?1 * @param lastname * @return */ List<Student> findByLastnameNot(String lastname); /** * … where x.age in ?1 * * @param ages * @return */ List<Student> findByAgeIn(Collection<Integer> ages); /** * … where x.age not in ?1 * * @param ages * @return */ List<Student> findByAgeNotIn(Collection<Integer> ages); List<Student> findByAgeNotInAndIdIn(Collection<Integer> ages, List<Integer> ids); /** * … where x.active = 1 * @return */ List<Student> findByActiveTrue(); /** * … where x.active = 0 * @return */ List<Student> findByActiveFalse(); /** * … where UPPER(x.firstname) = UPPER(?1) * @param firstname * @return */ List<Student> findByFirstnameIgnoreCase(String firstname); // 复杂的例子 List<Student> findByLastnameOrFirstnameAndIdBetweenOrderByAgeDescIdAsc(String lastname, String firstname, int id1, int id2); }
JPA查询使用注意事项:
- 方法名称必须findBy开头
- 数据库字段名必须遵从下划线模式,如:name, add_time,驼峰命名方式不支持JPA查询
关于fastmybatis
fastmybatis是一个mybatis开发框架,其宗旨为:简单、快速、有效。
- 零配置快速上手,无需依赖Spring
- 无需编写xml文件即可完成增删改查操作,支持LambdaQuery查询、支持JPA Query Method查询(findByXxx)
- 支持mysql、sqlserver、oracle、postgresql、sqlite、StarRocks(原DorisDB)
- 支持自定义sql,对于基本的增删改查不需要写SQL,对于其它特殊SQL(如统计SQL)可写在xml中
- 支持与spring-boot集成,依赖starter即可,支持Springboot3.0
- 支持插件编写
- 支持ActiveRecord模式
- 支持多租户
- 提供通用Service
- API丰富,多达40+方法,满足日常开发需求
- 轻量级,无侵入性,是官方mybatis的一种扩展
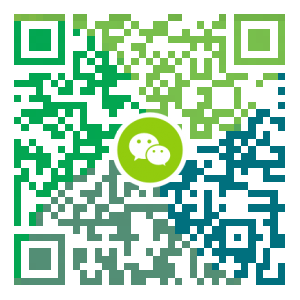
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
DriverBox V0.3 已发布
DriverBox V0.3 已发布 DriverBox是一款基于物联网开源框架Edgex打造的泛化协议接入服务。 以插件化的形式融合了 Modbus、Bacnet、HTTP、MQTT 等主流协议,同时也支持基于TCP的各类私有化协议对接。 我们期望DriverBox能够为相关人士提供更加高效、舒适的设备接入体验。 通过对各类设备的通信协议和数据交互形式进行抽象,定义了一套标准流程以涵盖泛化协议的共性处理逻辑,并结合动态解析脚本(Lua、Javascript、Python)填补其中的差异化部分。 以此解决设备接入过程中存在的驱动工程数量爆炸;接入标准难以规范化等问题。 版本更新 2023-07-06 v0.3 修复HTTP_Client、mqtt插件并发执行 lua 脚本问题 添加 config/update 接口,通过接口动态更新核心配置文件 简介 DriverBox是使用 Go(Golang)编写的边缘设备接入框架。通过配置接入设备,不需要写代码就可以接入绝大多数设备。 同时,DriverBox还提供了功能扩展,可以通过动态脚本(Lua)来实现非标准协议的适配和接入,大大提高了边缘...
- 下一篇
夜莺可观测性平台发布 v6.ga13 版本,正式版月底发布
What’s Changed feat: 告警信息支持通过 stdin 传递给 ibex 可以更方便地实现故障自愈 bytanxiao1990 feat: 心跳时间支持从 heartbeat 接口提取,提高了心跳时间实时性 byqifenggang feat: 登录密码支持 RSA 加密 bymasterjyq feat: 时序图表格模式 Legend 支持下钻链接 bymasterjyq refactor: 修改数据源类型和值后保存到 URL querystring refactor: 大盘详情页面标题设置为大盘标题 refactor: 大盘数据源变量支持正则过滤可选项 fix: 修复日志层级大于三层后字段名拼接错误问题 fix: 修复导入规则 group_id 错误问题 fix: 修复公开的大盘仍然会显示 row panel 编辑按钮问题 docs: 添加 TiDB 内置告警规则、仪表盘 bylongzhuquan docs: 增加 prometheus-operator、ipmi、kafka 内置告警规则和仪表盘 bycyancow 感谢相关 contributor 一起共建开源...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Windows10,CentOS7,CentOS8安装MongoDB4.0.16
- SpringBoot2全家桶,快速入门学习开发网站教程
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- Eclipse初始化配置,告别卡顿、闪退、编译时间过长
- Docker快速安装Oracle11G,搭建oracle11g学习环境
- 2048小游戏-低调大师作品
- SpringBoot2更换Tomcat为Jetty,小型站点的福音
- CentOS8,CentOS7,CentOS6编译安装Redis5.0.7
- CentOS7,8上快速安装Gitea,搭建Git服务器
- CentOS6,CentOS7官方镜像安装Oracle11G