Coap协议接入物联网平台(java实现)
概述
物联网平台支持CoAP协议连接通信。CoAP协议适用在资源受限的低功耗设备上,尤其是NB-IoT的设备使用。本文介绍基于开源的CoAP协议进行对称加密自主接入的流程,并提供java示例代码。
官方链接
流程
一、连接CoAP服务器
endpoint地址为:${YourProductKey}.coap.cn-shanghai.link.aliyuncs.com:${port}
${YourProductKey}:您的产品的ProductKey。
${port}:端口。使用对称加密时端口为5682。
———————————————————————————————————————
二、进行设备认证
具体请求参数详见官方文档
———————————————————————————————————————
三、上报数据
具体请求参数详见官方文档
示例代码
一、pom.xml依赖
<dependency> <groupId>org.eclipse.californium</groupId> <artifactId>californium-core</artifactId> <version>2.0.0-M17</version> </dependency> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.5</version> </dependency> <dependency> <groupId>commons-codec</groupId> <artifactId>commons-codec</artifactId> <version>1.13</version> </dependency> <dependency> <groupId>com.alibaba</groupId> <artifactId>fastjson</artifactId> <version>1.2.61</version> </dependency>
二、代码
/* * Copyright © 2019 Alibaba. All rights reserved. */ import java.io.IOException; import java.nio.charset.StandardCharsets; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.util.Set; import java.util.SortedMap; import java.util.TreeMap; import javax.crypto.Cipher; import javax.crypto.Mac; import javax.crypto.spec.IvParameterSpec; import javax.crypto.spec.SecretKeySpec; import org.apache.commons.codec.DecoderException; import org.apache.commons.codec.binary.Hex; import org.apache.commons.lang3.RandomUtils; import org.eclipse.californium.core.CoapClient; import org.eclipse.californium.core.CoapResponse; import org.eclipse.californium.core.Utils; import org.eclipse.californium.core.coap.CoAP; import org.eclipse.californium.core.coap.CoAP.Code; import org.eclipse.californium.core.coap.CoAP.Type; import org.eclipse.californium.core.coap.MediaTypeRegistry; import org.eclipse.californium.core.coap.Option; import org.eclipse.californium.core.coap.OptionNumberRegistry; import org.eclipse.californium.core.coap.OptionSet; import org.eclipse.californium.core.coap.Request; import org.eclipse.californium.elements.exception.ConnectorException; import com.alibaba.fastjson.JSONObject; /** * CoAP客户端连接阿里云物联网平台,基于eclipse californium开发。 * 自主接入开发流程及参数填写,请参见: * https://help.aliyun.com/document_detail/57697.html [使用对称加密自主接入] */ public class IotCoapClientWithAes { // ===================需要用户填写的参数,开始=========================== // 地域ID,当前仅支持华东2 private static String regionId = "cn-shanghai"; // 产品productKey private static String productKey = "a1fBBLKhpwv"; // 设备名成deviceName private static String deviceName = "AirC"; // 设备密钥deviceSecret private static String deviceSecret = "LL6VoF5L49EbMdoGvdwRppOgHWuwE9bL"; //发送的消息内容payload private static String payload = "hello coap!!"; // ===================需要用户填写的参数,结束=========================== // 定义加密方式 MAC算法可选以下多种算法 HmacMD5 HmacSHA1,需与signmethod一致。 private static final String HMAC_ALGORITHM = "hmacsha1"; // CoAP接入地址,对称加密端口号是5682。 private static String serverURI = "coap://" + productKey + ".coap." + regionId + ".link.aliyuncs.com:5682"; // 发送消息用的Topic。需要在控制台自定义Topic,设备操作权限需选择为“发布”。 private static String updateTopic = "/" + productKey + "/" + deviceName + "/user/update"; // token option private static final int COAP2_OPTION_TOKEN = 2088; // seq option private static final int COAP2_OPTION_SEQ = 2089; // 加密算法sha256 private static final String SHA_256 = "SHA-256"; private static final int DIGITAL_16 = 16; private static final int DIGITAL_48 = 48; // CoAP客户端 private CoapClient coapClient = new CoapClient(); // token 7天有效,失效后需要重新获取。 private String token = null; private String random = null; @SuppressWarnings("unused") private long seqOffset = 0; /** * 初始化CoAP客户端 * * @param productKey 产品key * @param deviceName 设备名称 * @param deviceSecret 设备密钥 */ public void conenct(String productKey, String deviceName, String deviceSecret) { try { // 认证uri,/auth String uri = serverURI + "/auth"; // 只支持POST方法 Request request = new Request(Code.POST, Type.CON); // 设置option OptionSet optionSet = new OptionSet(); optionSet.addOption(new Option(OptionNumberRegistry.CONTENT_FORMAT, MediaTypeRegistry.APPLICATION_JSON)); optionSet.addOption(new Option(OptionNumberRegistry.ACCEPT, MediaTypeRegistry.APPLICATION_JSON)); request.setOptions(optionSet); // 设置认证uri request.setURI(uri); // 设置认证请求payload request.setPayload(authBody(productKey, deviceName, deviceSecret)); // 发送认证请求 CoapResponse response = coapClient.advanced(request); System.out.println(Utils.prettyPrint(response)); System.out.println(); // 解析请求响应 JSONObject json = JSONObject.parseObject(response.getResponseText()); token = json.getString("token"); random = json.getString("random"); seqOffset = json.getLongValue("seqOffset"); } catch (ConnectorException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } /** * 发送消息 * * @param topic 发送消息的Topic * @param payload 消息内容 */ public void publish(String topic, byte[] payload) { try { // 消息发布uri,/topic/${topic} String uri = serverURI + "/topic" + topic; // AES加密seq,seq=RandomUtils.nextInt() String shaKey = encod(deviceSecret + "," + random); byte[] keys = Hex.decodeHex(shaKey.substring(DIGITAL_16, DIGITAL_48)); byte[] seqBytes = encrypt(String.valueOf(RandomUtils.nextInt()).getBytes(StandardCharsets.UTF_8), keys); // 只支持POST方法 Request request = new Request(CoAP.Code.POST, CoAP.Type.CON); // 设置option OptionSet optionSet = new OptionSet(); optionSet.addOption(new Option(OptionNumberRegistry.CONTENT_FORMAT, MediaTypeRegistry.APPLICATION_JSON)); optionSet.addOption(new Option(OptionNumberRegistry.ACCEPT, MediaTypeRegistry.APPLICATION_JSON)); optionSet.addOption(new Option(COAP2_OPTION_TOKEN, token)); optionSet.addOption(new Option(COAP2_OPTION_SEQ, seqBytes)); request.setOptions(optionSet); // 设置消息发布uri request.setURI(uri); // 设置消息payload request.setPayload(encrypt(payload, keys)); // 发送消息 CoapResponse response = coapClient.advanced(request); System.out.println("----------------"); System.out.println(request.getPayload().length); System.out.println("----------------"); System.out.println(Utils.prettyPrint(response)); // 解析消息发送结果 String result = null; if (response.getPayload() != null) { result = new String(decrypt(response.getPayload(), keys)); } System.out.println("payload: " + result); System.out.println(); } catch (ConnectorException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } catch (DecoderException e) { e.printStackTrace(); } } /** * 生成认证请求内容 * * @param productKey 产品key * @param deviceName 设备名字 * @param deviceSecret 设备密钥 * @return 认证请求 */ private String authBody(String productKey, String deviceName, String deviceSecret) { // 构建认证请求 JSONObject body = new JSONObject(); body.put("productKey", productKey); body.put("deviceName", deviceName); body.put("clientId", productKey + "." + deviceName); body.put("timestamp", String.valueOf(System.currentTimeMillis())); body.put("signmethod", HMAC_ALGORITHM); body.put("seq", DIGITAL_16); body.put("sign", sign(body, deviceSecret)); System.out.println("----- auth body -----"); System.out.println(body.toJSONString()); return body.toJSONString(); } /** * 设备端签名 * * @param params 签名参数 * @param deviceSecret 设备密钥 * @return 签名十六进制字符串 */ private String sign(JSONObject params, String deviceSecret) { // 请求参数按字典顺序排序 Set<String> keys = getSortedKeys(params); // sign、signmethod、version、resources除外 keys.remove("sign"); keys.remove("signmethod"); keys.remove("version"); keys.remove("resources"); // 组装签名明文 StringBuffer content = new StringBuffer(); for (String key : keys) { content.append(key); content.append(params.getString(key)); } // 计算签名 String sign = encrypt(content.toString(), deviceSecret); System.out.println("sign content=" + content); System.out.println("sign result=" + sign); return sign; } /** * 获取JSON对象排序后的key集合 * * @param json 需要排序的JSON对象 * @return 排序后的key集合 */ private Set<String> getSortedKeys(JSONObject json) { SortedMap<String, String> map = new TreeMap<String, String>(); for (String key : json.keySet()) { String vlaue = json.getString(key); map.put(key, vlaue); } return map.keySet(); } /** * 使用 HMAC_ALGORITHM 加密 * * @param content 明文 * @param secret 密钥 * @return 密文 */ private String encrypt(String content, String secret) { try { byte[] text = content.getBytes(StandardCharsets.UTF_8); byte[] key = secret.getBytes(StandardCharsets.UTF_8); SecretKeySpec secretKey = new SecretKeySpec(key, HMAC_ALGORITHM); Mac mac = Mac.getInstance(secretKey.getAlgorithm()); mac.init(secretKey); return Hex.encodeHexString(mac.doFinal(text)); } catch (Exception e) { e.printStackTrace(); return null; } } /** * SHA-256 * * @param str 待加密的报文 */ private String encod(String str) { MessageDigest messageDigest; String encdeStr = ""; try { messageDigest = MessageDigest.getInstance(SHA_256); byte[] hash = messageDigest.digest(str.getBytes(StandardCharsets.UTF_8)); encdeStr = Hex.encodeHexString(hash); } catch (NoSuchAlgorithmException e) { System.out.println(String.format("Exception@encod: str=%s;", str)); e.printStackTrace(); return null; } return encdeStr; } // AES 加解密算法 private static final String IV = "543yhjy97ae7fyfg"; private static final String TRANSFORM = "AES/CBC/PKCS5Padding"; private static final String ALGORITHM = "AES"; /** * key length = 16 bits */ private byte[] encrypt(byte[] content, byte[] key) { return encrypt(content, key, IV); } /** * key length = 16 bits */ private byte[] decrypt(byte[] content, byte[] key) { return decrypt(content, key, IV); } /** * aes 128 cbc key length = 16 bits */ private byte[] encrypt(byte[] content, byte[] key, String ivContent) { try { SecretKeySpec keySpec = new SecretKeySpec(key, ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORM); IvParameterSpec iv = new IvParameterSpec(ivContent.getBytes(StandardCharsets.UTF_8)); cipher.init(Cipher.ENCRYPT_MODE, keySpec, iv); return cipher.doFinal(content); } catch (Exception ex) { System.out.println( String.format("AES encrypt error, %s, %s, %s", content, Hex.encodeHex(key), ex.getMessage())); return null; } } /** * aes 128 cbc key length = 16 bits */ private byte[] decrypt(byte[] content, byte[] key, String ivContent) { try { SecretKeySpec keySpec = new SecretKeySpec(key, ALGORITHM); Cipher cipher = Cipher.getInstance(TRANSFORM); IvParameterSpec iv = new IvParameterSpec(ivContent.getBytes(StandardCharsets.UTF_8)); cipher.init(Cipher.DECRYPT_MODE, keySpec, iv); return cipher.doFinal(content); } catch (Exception ex) { System.out.println(String.format("AES decrypt error, %s, %s, %s", Hex.encodeHex(content), Hex.encodeHex(key), ex.getMessage())); return null; } } public static void main(String[] args) throws InterruptedException { IotCoapClientWithAes client = new IotCoapClientWithAes(); client.conenct(productKey, deviceName, deviceSecret); client.publish(updateTopic, payload.getBytes(StandardCharsets.UTF_8)); } }
注意事项
1、coap协议是短连接,和mqtt长连接不同,所以在控制台的设备行为分析日志里看不到记录,需要注意。
2、coap协议发送的payload有大小限制,不能超过1KB,如果超过的话这个请求会被拒绝,消息发不到平台。代码中System.out.println(request.getPayload().length);就是用来打印payload长度的。
3、目前只有华东2地域的设备支持使用coap协议接入。
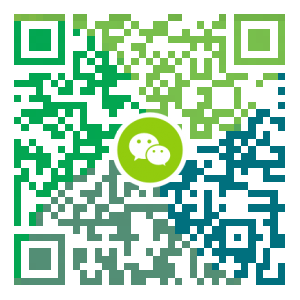
低调大师中文资讯倾力打造互联网数据资讯、行业资源、电子商务、移动互联网、网络营销平台。
持续更新报道IT业界、互联网、市场资讯、驱动更新,是最及时权威的产业资讯及硬件资讯报道平台。
转载内容版权归作者及来源网站所有,本站原创内容转载请注明来源。
- 上一篇
手把手教你用Spuernova生成flutter代码
作者:Keriy链接:https://juejin.im/post/5e1c5d7ef265da3df860f9bf来源:掘金 缘起 周末得空,逛了dribbble,发现了好多好看的设计,馋的不行。相信每个前端都有这样一个梦想:能把设计稿直接生成代码该多好,忽而想起了Flutter Interact上大佬们演示的插件,感觉有得搞? sketch准备 没有vip不能下载,就自己照着预览图画一个,丑莫怪~ Spuernova or xd-to-flutter xd-to-flutter 在我准备安装的时候,得到了这样的提示: 呵呵~ 好在Spuernova(这货是收费的,哈哈哈)可以同时支持XD和sketch,那么话不多说,下载安装,导入 这里直接选择全部页面 搞定~,so easy 生成的代码可以直接点击右上角的到处图标到处成项目或者单个文件, 这样就完工啦~ 生成的项目结构大致如下: 运行 生成的代码在安装完成后可以直接运行。用VSCode打开刚刚生成的项目,flutter pub get一波没有问题, flutter run起来看看 海星,感觉哪里不对?字体图标和字体怎么都这样样子...
- 下一篇
阿里云IoT Studio服务开发操作OSS存储服务示例
概述 IoT Studio服务开发是一个物联网业务逻辑的开发工具,通过编排服务节点的方式快速完成简单的物联网业务逻辑的设计。本文演示如何使用NodeJS节点基于OSS NodeJS SDK操作阿里云OSS存储服务。 Step By Step 1、拖拽服务开发控件 2、安装ali-oss 3、编写NodeJs脚本 /** * @param {Object} payload 上一节点的输出 * @param {Object} node 指定某个节点的输出 * @param {Object} query 服务流第一个节点的输出 * @param {Object} context { appKey, appSecret } */ module.exports = async function(payload, node, query, context) { // const result; let OSS = require('ali-oss'); // region https://help.aliyun.com/document_detail/31837.html?spm=a2c4g.11...
相关文章
文章评论
共有0条评论来说两句吧...
文章二维码
点击排行
推荐阅读
最新文章
- Mario游戏-低调大师作品
- CentOS关闭SELinux安全模块
- CentOS7,CentOS8安装Elasticsearch6.8.6
- CentOS8安装MyCat,轻松搞定数据库的读写分离、垂直分库、水平分库
- Docker安装Oracle12C,快速搭建Oracle学习环境
- Red5直播服务器,属于Java语言的直播服务器
- CentOS8编译安装MySQL8.0.19
- SpringBoot2整合MyBatis,连接MySql数据库做增删改查操作
- Springboot2将连接池hikari替换为druid,体验最强大的数据库连接池
- CentOS6,CentOS7官方镜像安装Oracle11G